The Select component has support to render default html select with slot or options prop. You can customize the option component for one or all options. See Examples.
1<x-select2 label="Search a User"3 wire:model.defer="model"4 placeholder="Select some user"5 :async-data="route('api.users.index')"6 option-label="name"7 option-value="id"8/>
WireUi will make a request with the search parameter(string) when the user types in the input.
When the component is initialized and has any selected value,
the select will send a request with the selected parameter(array) to find the selected option.
You are free to create your API as you want, just apply these two scopes: search and selected
You can customize the asyncData prop to change the http method and add more data to the request.
1export type AsyncDataConfig = {2 api: string | null3 method: string4 params: any,5 credentials?: RequestCredentials,6 alwaysFetch: boolean7}
How to Customize the async-data?
The queryString params will be merged with the asyncData params
1<x-select ... :async-data="route('api.users.index', ['foo' => 'bar'])" /> 2 3OR 4 5<x-select ... :async-data="[ 6 'api' => route('api.users.index'), 7 'method' => 'POST', // default is GET 8 'params' => ['ble' => 'baz'], // default is [] 9 'credentials' => 'include' // default is undefined10]" />
1<x-select2 label="Select Status"3 placeholder="Select one status"4 :options="['Active', 'Pending', 'Stuck', 'Done']"5 wire:model.defer="model"6/>
1<x-select2 label="Select Statuses"3 placeholder="Select many statuses"4 multiselect5 :options="['Active', 'Pending', 'Stuck', 'Done']"6 wire:model.defer="model"7/>
1<x-select 2 label="Select Status" 3 placeholder="Select one status" 4 :options="[ 5 ['name' => 'Active', 'id' => 1], 6 ['name' => 'Pending', 'id' => 2], 7 ['name' => 'Stuck', 'id' => 3], 8 ['name' => 'Done', 'id' => 4], 9 ]"10 option-label="name"11 option-value="id"12 wire:model.defer="model"13/>
1<x-select 2 label="Select Status" 3 placeholder="Select one status" 4 :options="[ 5 ['name' => 'Active', 'id' => 1, 'description' => 'The status is active'], 6 ['name' => 'Pending', 'id' => 2, 'description' => 'The status is pending'], 7 ['name' => 'Stuck', 'id' => 3, 'description' => 'The status is stuck'], 8 ['name' => 'Done', 'id' => 4, 'description' => 'The status is done'], 9 ]"10 option-label="name"11 option-value="id"12 wire:model.defer="model"13/>
1<x-select 2 label="Select Status" 3 placeholder="Select one status" 4 wire:model.defer="model" 5> 6 <x-select.option label="Pending" value="1" /> 7 <x-select.option label="In Progress" value="2" /> 8 <x-select.option label="Stuck" value="3" /> 9 <x-select.option label="Done" value="4" />10</x-select>
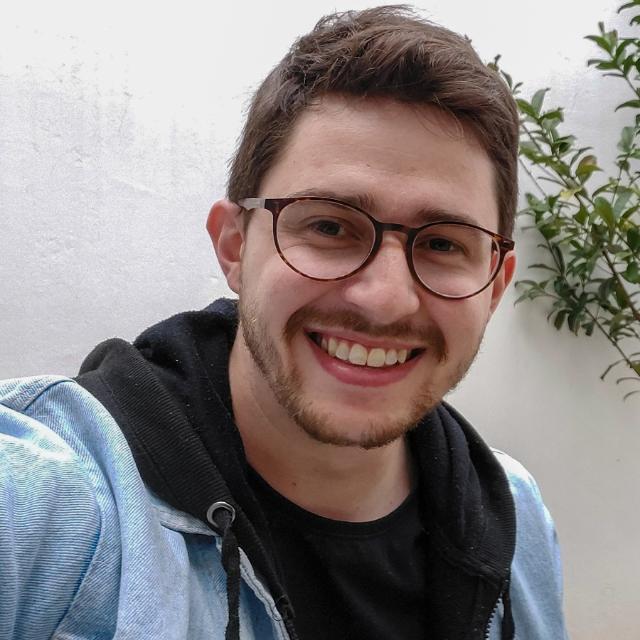
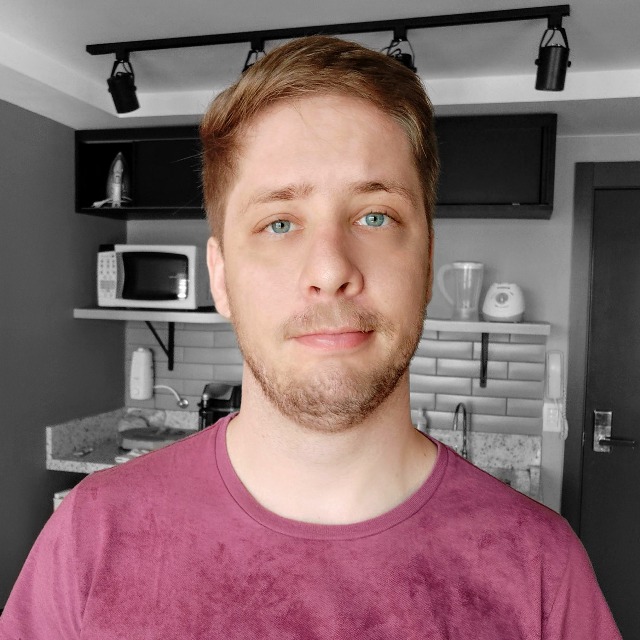
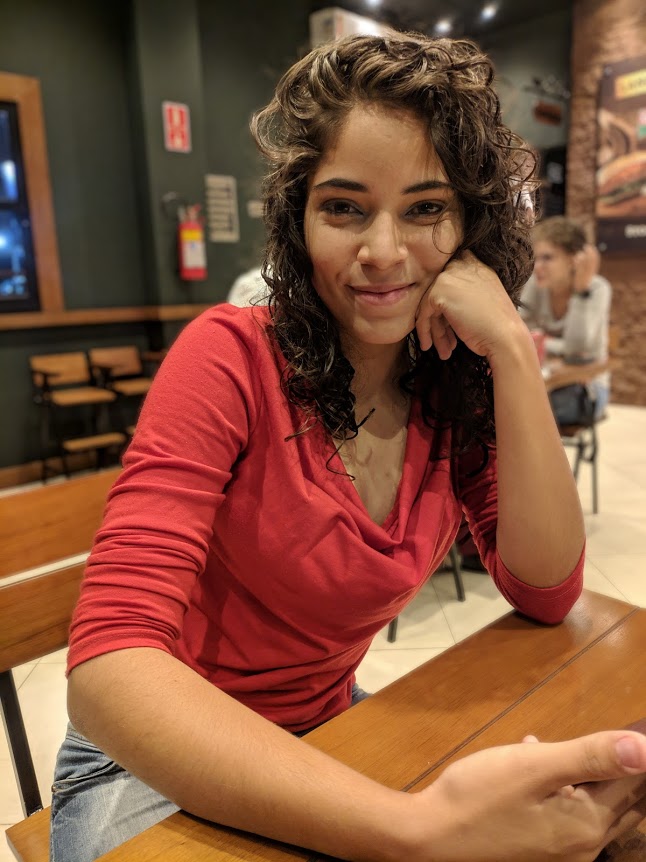
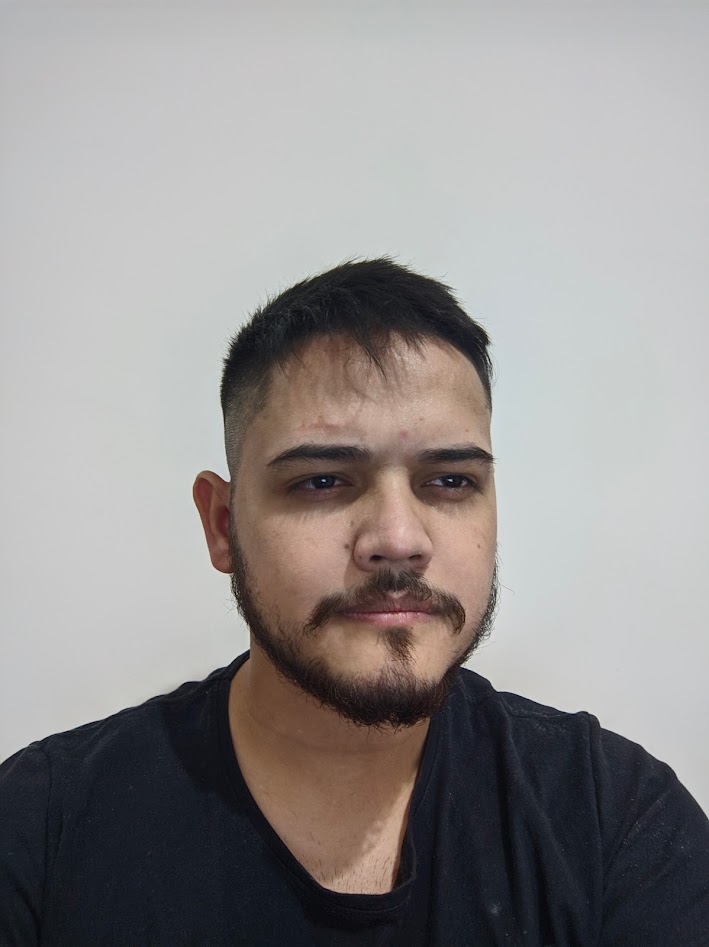
1<div class="grid grid-cols-1 sm:grid-cols-2 gap-5 sm:max-w-lg"> 2 <x-select 3 label="Select Relator" 4 placeholder="Select relator" 5 wire:model.defer="model" 6 > 7 <x-select.user-option src="https://via.placeholder.com/500" label="People 1" value="1" /> 8 <x-select.user-option src="https://via.placeholder.com/500" label="People 2" value="2" /> 9 <x-select.user-option src="https://via.placeholder.com/500" label="People 3" value="3" />10 <x-select.user-option src="https://via.placeholder.com/500" label="People 4" value="4" />11 </x-select>12 13 <x-select14 label="Search a User"15 wire:model.defer="asyncSearchUser"16 placeholder="Select some user"17 :async-data="route('api.users.index')"18 :template="[19 'name' => 'user-option',20 'config' => ['src' => 'profile_image']21 ]"22 option-label="name"23 option-value="id"24 option-description="email"25 />26</div>
Options
Prop | Required | Default | Type | Available |
---|---|---|---|---|
label | false | none | string | * |
placeholder | false | none | string | * |
option-value | false | none | string | * |
option-label | false | none | string | * |
option-description | false | none | string | * |
options | false | none | Collection|array | * |
flip-options | false | false | boolean | |
option-key-value | false | false | boolean | |
clearable | false | true | boolean | boolean |
searchable | false | true | boolean | boolean |
multiselect | false | false | boolean | boolean |
icon | false | none | string | all heroicons |
rightIcon | false | selector | string | all heroicons |
async-data | false | none | string|AsyncDataConfig | all endpoints |
always-fetch | false | none | string | true|false |
template | false | default | string|array | default|user-option |
empty-message | false | __('wireui::messages.empty_options') | string | * |
hide-empty-message | false | false | boolean | boolean |
min-items-for-search | false | 11 | int | * |
Options
Prop | Required | Default | Type |
---|---|---|---|
description | false | none | string |
label | false | none | string|null |
value | true | none | ayn |
readonly | false | false | boolean |
disabled | false | false | boolean |
option | false | none | Model|stdClass|array|null |
Options
Prop | Required | Default | Type |
---|---|---|---|
description | false | none | string |
label | true | none | string|null |
value | true | none | any |
readonly | false | false | boolean |
disabled | false | false | boolean |
src | true | none | string|null |
option | true | none | Model|stdClass|array|null |
1<x-select2 ...3 x-on:open="alert('openned select')"4 x-on:close="alert('closed select')"5 x-on:selected="alert('selected/unselected option')"6 x-on:clear="alert('cleared select')"7>8 ...9</x-select>
1<x-select 2 label="Search a User" 3 wire:model.defer="model" 4 placeholder="Select some user" 5 :async-data="route('api.users.index')" 6 option-label="name" 7 option-value="id" 8 hide-empty-message 9>10 <x-slot name="afterOptions" class="p-2 flex justify-center" x-show="displayOptions.length === 0">11 <x-button12 x-on:click="close"13 primary14 flat15 full>16 <span x-html="`Create user <b>${search}</b>`"></span>17 </x-button>18 </x-slot>19</x-select>
1<x-slot name="beforeOptions">2 // html code3</x-slot>4 5<x-slot name="afterOptions">6 // html code7</x-slot>